すぐに使えるVue3 + Vite2 + TypeScriptなど。 大規模なアプリケーションを迅速に構築するためのテンプレートフレームワーク。 さまざまなプラグインが統合され、モジュール化とリードオンデマンド用に最適化されているため、自信を持って使用できます。 [ドキュメントを更新するには、ここをクリックしてください](https://github.com/tobe-fe-dalao/fast-vue3/blob/main/docs/update.md)
[English](./README.md) | [简体中文](./README-zh_CN.md) | 日本語
# 特徴
ここでは、いくつかのコアパーツの簡単な紹介を示しますが、インストールパーツについては詳しく説明しません。 公式ドキュメントまたは[ビジュアルウェアハウス](https://github1s.com/tobe-fe-dalao/fast-vue3)を直接読むことをお勧めします。
## 🪂技術巨人のコラボレーション-コード仕様
🪁 現在、多くのハイテク巨人チームは、一般的に [husky](https://github.com/typicode/husky) と [lint-staged](https://github.com/okonet/lint-staged)を使用してコード仕様を制約しています。
- `pre-commit`を介して、lintチェック、単体テスト、コードフォーマットなどを実装します。
- VsCodeと組み合わせる(保存時に自動的にフォーマットする:editor.formatOnSave:true)
- Gitフックと組み合わせる(コミット前に実行:pre-commit => npm run lint:lint-staged)
- IDE構成( `.editorconfig`)、ESLint構成(` .eslintrc.js`和 `.eslintignore`)、StyleLint構成(` .stylelintrc`和 `.stylelintignore`)、詳細については、対応する構成ファイルを参照してください.
🔌 コード仕様を閉じる
`.eslintignore` と ` .stylelintignore`をそれぞれ `src /`ディレクトリに追加して無視します.
## ディレクトリ構造
システムのディレクトリ構造は次のとおりです
```
├── config
│ ├── vite // vite 構成
│ ├── constant // システム定数
| └── themeConfig // theme 構成
├── docs // ドキュメント関連
├── mock // モックデータ
├── plop-tpls // plopテンプレート
├── src
│ ├── api // APIリクエスト
│ ├── assets // 静的ファイル
│ ├── components // コンポーネント
│ ├── page // ページ
│ ├── router // ルーティングファイル
│ ├── store // 状態管理
│ ├── utils // ツール
│ ├── App.vue // vue テンプレート エントリ
│ ├── main.ts // vue テンプレート js
├── .d.ts // タイプ定義
├── tailwind.config.js // tailwind グローバル構成
├── tsconfig.json // ts 構成
└── vite.config.ts // vite グローバル構成
```
## 💕JSX構文をサポートする
```json
{
...
"@vitejs/plugin-vue-jsx": "^1.3.3"
...
}
```
## 🎸 UIコンポーネントはオンデマンドで読み込まれ、自動的にインポートされます
```typescript
// モジュラーライティング
import Components from 'unplugin-vue-components/vite'
export const AutoRegistryComponents = () => {
return Components({
extensions: ['vue', 'md'],
deep: true,
dts: 'src/components.d.ts',
directoryAsNamespace: false,
globalNamespaces: [],
directives: true,
include: [/\.vue$/, /\.vue\?vue/, /\.md$/],
exclude: [/[\\/]node_modules[\\/]/, /[\\/]\.git[\\/]/, /[\\/]\.nuxt[\\/]/],
resolvers: [
IconsResolver({
componentPrefix: '',
}),
ArcoResolver({ importStyle: 'less' }),// 必要に応じてUIフレームワークを追加します
VueUseComponentsResolver(),// VueUseコンポーネントがデフォルトで使用されます
],
})
}
```
## 🧩Viteプラグインのモジュール性
プラグインの管理を容易にするために、すべての `config`を` config / vite / plugins`に入れてください。 将来的には、非常にクリーンに管理するために、フォルダーに直接分割されたプラグインが増える予定です。
`Fast-Vue3`は、特定のプラグインの動的なオープンを区別するために、統合された環境変数管理を追加することは言及する価値があります。
```typescript
// vite/plugins/index.ts
/**
* @name createVitePlugins
* @description Encapsulate the plugins array to call uniformly
*/
import type { Plugin } from 'vite';
import vue from '@vitejs/plugin-vue';
import vueJsx from '@vitejs/plugin-vue-jsx';
import { ConfigSvgIconsPlugin } from './svgIcons';
import { AutoRegistryComponents } from './component';
import { AutoImportDeps } from './autoImport';
import { ConfigMockPlugin } from './mock';
import { ConfigVisualizerConfig } from './visualizer';
import { ConfigCompressPlugin } from './compress';
import { ConfigPagesPlugin } from './pages'
import { ConfigMarkDownPlugin } from './markdown'
import { ConfigRestartPlugin } from './restart'
export function createVitePlugins(isBuild: boolean) {
const vitePlugins: (Plugin | Plugin[])[] = [
// vueサポート
vue(),
// JSXサポート
vueJsx(),
// コンポーネントをオンデマンドで自動的にインポート
AutoRegistryComponents(),
// 必要に応じて依存関係を自動的にインポートします
AutoImportDeps(),
// ルートを自動的に生成する
ConfigPagesPlugin(),
// .gz圧縮を有効にする rollup-plugin-gzip
ConfigCompressPlugin(),
// markdownサポート
ConfigMarkDownPlugin(),
// 構成ファイルの変更を監視して再起動します
ConfigRestartPlugin(),
];
// vite-plugin-svg-icons
vitePlugins.push(ConfigSvgIconsPlugin(isBuild));
// vite-plugin-mock
vitePlugins.push(ConfigMockPlugin(isBuild));
// rollup-plugin-visualizer
vitePlugins.push(ConfigVisualizerConfig());
return vitePlugins;
}
```
`vite.config.ts` is much cleaner
```typescript
import { createVitePlugins } from './config/vite/plugins'
...
return {
resolve: {
alias: {
"@": path.resolve(__dirname, './src'),
'@config': path.resolve(__dirname, './config'),
"@components": path.resolve(__dirname, './src/components'),
'@utils': path.resolve(__dirname, './src/utils'),
'@api': path.resolve(__dirname, './src/api'),
}
},
// plugins
plugins: createVitePlugins(isBuild)
}
...
```
## 📱 Support for `Pinia`, the next generation of `Vuex5`
ファイルを作成する `src/store/index.ts`
```typescript
// モジュール化をサポートし、plopを使用してコマンドラインからワンクリックで生成できます
import { createPinia } from 'pinia';
import { useAppStore } from './modules/app';
import { useUserStore } from './modules/user';
const pinia = createPinia();
export { useAppStore, useUserStore };
export default pinia;
```
ファイルを作成する `src/store/modules/user/index.ts`
```typescript
import { defineStore } from 'pinia'
import piniaStore from '@/store'
export const useUserStore = defineStore(
// unique id
'user',
{
state: () => ({}),
getters: {},
actions: {}
}
)
```
## 🤖 ファイルを自動的に生成するための `Plop`をサポート
⚙️ コードファイルは自動的に生成され、3つのプリセットテンプレート `pages`、` components`、 `store`を提供します。また、必要に応じて、より多くの自動生成スクリプトを設計することもできます。 通常、バックエンドエンジニアはこのフォームを使用します。これは非常に効率的です。。
```shell
# install plop
pnpm add plop
```
ルートディレクトリ `plopfile.ts`に作成します
```typescript
import { NodePlopAPI } from 'plop';
export default function (plop: NodePlopAPI) {
plop.setWelcomeMessage('Please select the pattern you want to create')
plop.setGenerator('page', require('./plop-tpls/page/prompt'))
plop.setGenerator('component', require('./plop-tpls/component/prompt'))
plop.setGenerator('store', require('./plop-tpls/store/prompt'))
}
```
```shell
# start command
pnpm run plop
```
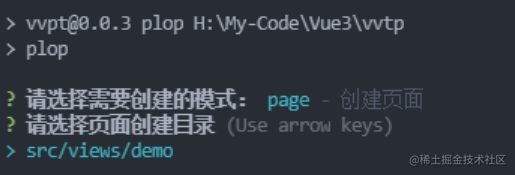
## 🖼️ `SVG`アイコンのサポート
ブラウザの互換性の向上に伴い、SVGのパフォーマンスは徐々に顕著になりました。 多くの技術大手チームが独自のSVG管理ライブラリを作成しており、ツールライブラリは後で推奨されます。
```shell
# svg依存関係をインストールします
pnpm add vite-plugin-svg-icons
```
設定 `vite.config.ts`
```typescript
import viteSvgIcons from 'vite-plugin-svg-icons';
export default defineConfig({
plugins:[
...
viteSvgIcons({
// キャッシュする必要のあるアイコンフォルダを指定します
iconDirs: [path.resolve(process.cwd(), 'src/assets/icons')],
// symbolId形式を指定します
symbolId: 'icon-[dir]-[name]',
}),
]
...
})
```
単純な `SvgIcon`コンポーネントがカプセル化されており、ファイルの下の` svg`を直接読み取ることができ、フォルダーディレクトリに従ってファイルを自動的に見つけることができます。
```html
```
## 📦 サポート `axios (ts version)`
主流のインターセプター、リクエスト呼び出し、その他のメソッドをカプセル化し、モジュール `index.ts` /` status.ts` / `type.ts`を区別しています。
```typescript
// カプセル化 src/api/user/index.ts
import request from '@utils/http/axios'
import { IResponse } from '@utils/http/axios/type'
import { ReqAuth, ReqParams, ResResult } from './type';
enum URL {
login = '/v1/user/login',
userProfile = 'mock/api/userProfile'
}
const getUserProfile = async () => request